Solution to the Dining Philosophers Problem
View on GitHub →Implemented the dining philosophers problem to get my head around thread synchronization without boring myself to death. but actually watching virtual philosophers starve is more entertaining than reading textbook explanations.
The Setup:
- Philosophers sitting at a table thinking deep thoughts (sleeping)
- Forks between them that they need to grab to eat
- A perfect recipe for deadlock when everyone grabs one fork and waits forever
Solved it three different ways because I’m indecisive:
- Resource hierarchy approach - philosophers grab the lower-numbered fork first, breaking the cycle that causes deadlock
- “Waiter” solution using a monitor to ensure at most N-1 philosophers try to eat at once
- Timed attempts with backoff when things get stuck
Added a terminal visualization that’s oddly mesmerizing to watch - philosophers transition between thinking, hungry, and eating states while forks change hands.
The hierarchy solution ended up being the cleanest, but the waiter approach had better throughput. Timed attempts worked but introduced the possibility of starvation (ironically appropriate for philosophers).
Started this to understand mutex locking patterns and ended up spending way too much time optimizing for “fork fairness” - ensuring no philosopher waits significantly longer than others. Classic yak shaving, but I finally understand why concurrency is hard.
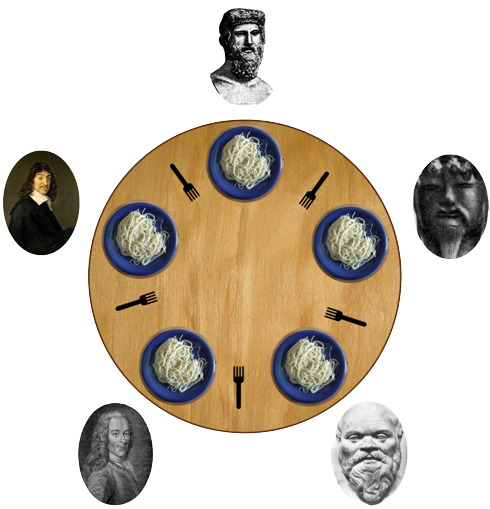